Reading Time: 5 minutes read
I had much too hard of a time groking the Figma Plugin’s documentation, and thought I would leave a note for any brave souls to follow.
Figma has a great API and documentation around how to make a plugin on the desktop app. When writing a plugin, you have access to the entire Figma canvas of the active file, to which you can read/write content. You also have quite a lenient window API from which you can make external requests and do things such as download assets or OAuth into services.
All of this being said, you are best off learning about what you can do directly from Figma here.
If you are like me, and working on a plugin, which you have decided to write in React, then you may encounter the desire to receive callback events from the Figma canvas in your app. In my case, I wanted the React application to react to the updated user selection, such that I could access the content of a TextNode, and update the plugin content accordingly.
To do this, I struggled with the Figma Plugin examples to understand how to access data from the canvas and into my app. The Figma Plugin examples, which can be found here, have a React application sample which sends data to the canvas, but not the other way around. While this is seemingly straight forward, I didn’t immediately absorb the explanations from Figma Plugin website.
In retrospect, the way to do this is quite simple.
First, the Figma Plugin API uses the Window postMessage API to transmit information. This is explained in the Plugin documentation with a clear diagram which you can see here:
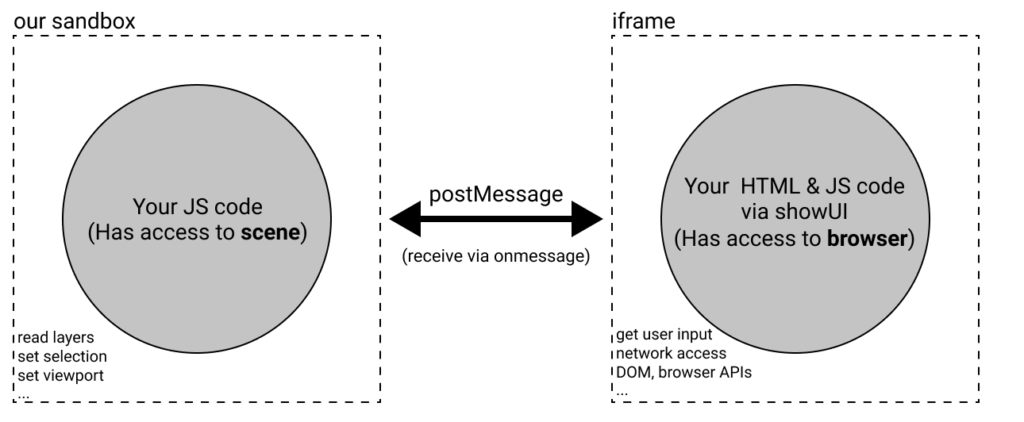
The first thing to note from this diagram is the postMessage API, which I mentioned above. The second thing is that the postMessage API is bi-directional, and allows for data to go from the app to the canvas, and vice-versa.
Practically speaking, the React Figma Plugin demo shows this in the example
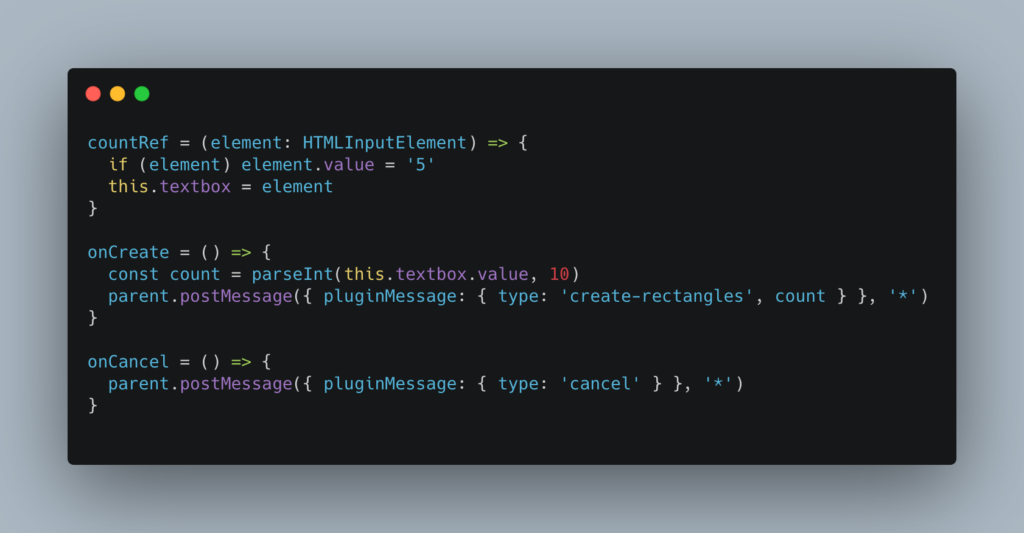
In the example, the postMessage API is using the window.parent
object to announce from the React app to the Figma canvas. Specifically, from the plugin example, there are two JavaScript files – code.ts
and ui.tsx
, which respectively handle the code that directly manages the figma
plugin API, and the UI code for the plugin itself.
While the parent
object is used to send data to the canvas, you need to do something different to receive data. You can learn about how the window.parent API exists here. In short, iFrames can speak to the parent windows. As the Figma Plugin ecosystem runs in a iFrame, this is how the postMessages are exchanged.
To receive data from the figma
api, you need to setup a postMessage from the code.ts
file, which has access to the figma
object.
In my case, the example is that I would like to access the latest selected items from the figma canvas, when the user has selected something new. To do that, I have the following code which creates an event listener on the figma
object, and then broadcasts a postMessage containing that information.
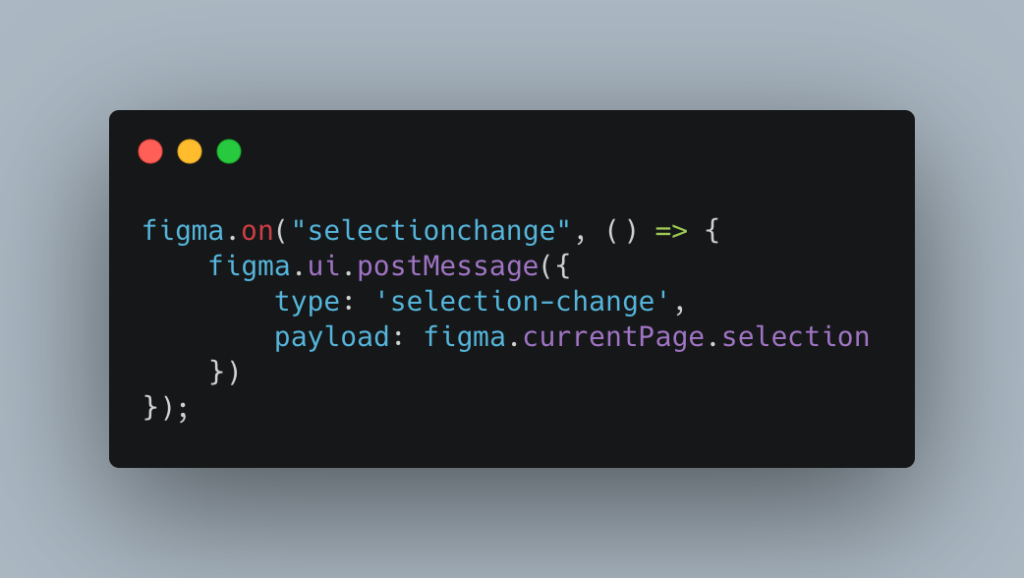
Once the figma object broadcasts the message, the React app can then receive the message. To receive this from the React application, you can create a simple EventListener on message
.
Now the part that was unintuitive, given the example, was that the React app listens directly to the window object to receive the data broadcasted from the code.ts
file. You can see an example below.
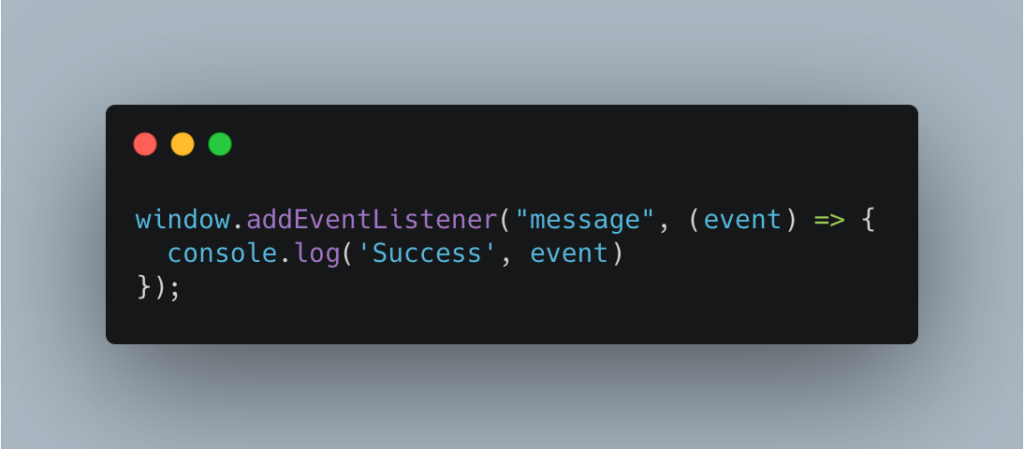
As you can see, to listen for the event in the React application, the window.addEventListener
is used, as opposed to parent.addEventListener
. This is done because the React application is unable to setup event listeners on the parent
, due to cross-origin rules. To bypass this, you can access the window
object, and the postMessage API properly passes the data that was broadcasted from the code.ts
file.
To summarize, to get data from the React application to the Figma plugin, you use parent.postMessage
in your React code, which is demo-ed as the ui.tsx
file. To get data from the Figma canvas into the React application, you need to broadcast a postMessage
message using the figma.ui.postMessage
method (demo-ed from code.ts
), which then can be listened to from the React application using the window.addEventListener
.
I hope this helps if you are looking to send data from the Figma Plugin to your React application!